Ví dụ này mình sẽ hướng dẫn các bạn sử dụng SwipeRefreshLayout với RecyclerView trong ứng dụng Android.
Để có thể luôn hiển thị những dữ liệu mới nhất, chúng ta thường thực hiện cập nhật dữ liệu một cách tự động trong 1 thời gian ngắn hoặc 1 thời gia khá dài, trong nhiều trường hợp đó không phải hoàn toàn là một cách hay từ phía user, nó có thể gây tiêu tốn lưu lượng mà khiến user thấy đó là không cần thiết hoặc cũng có thể khiến user thấy lâu có hàng mới thế. Vì vậy sao ta ko để user có thể tự mình cập nhật dữ liệu mới khi thấy cần thiết. Và thật hay ho là nền tảng Android cung cấp cho chúng ta một design pattern swipe-to-refresh để thực hiện việc đó.
Chú ý: Lớp này yêu cần phiên bản mới nhất của Android v4 Support Library APIs.
Ví dụ đối với các ứng dụng thời tiết, khi user muốn biết bây giờ lạnh bao nhiêu độ thì chỉ đơn giản là kéo ngón tay từ top màn hình xuống để refresh data, thật tiện lợi phải không.
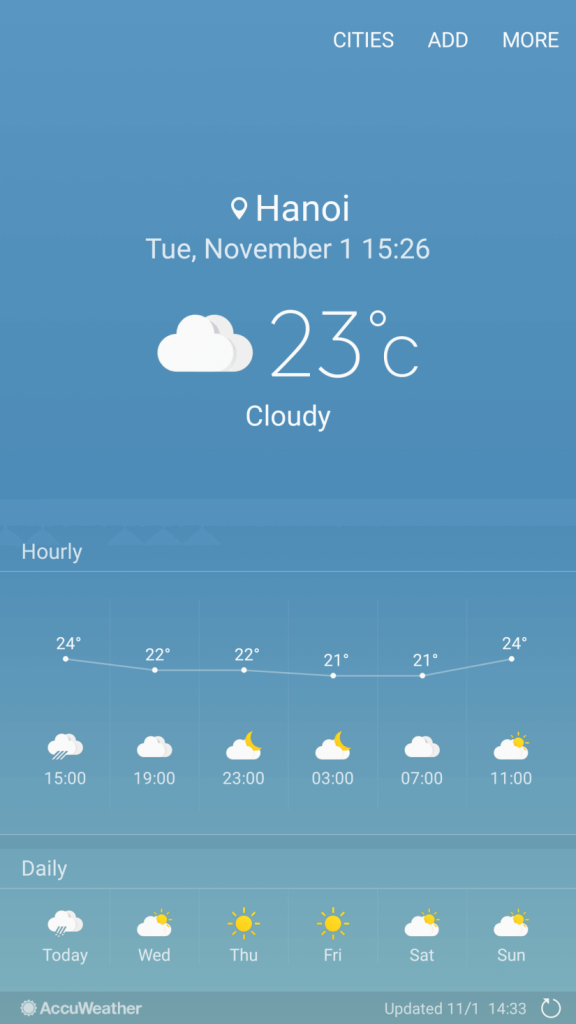
Add the SwipeRefreshLayout Widget
Add SwipeRefreshLayout như là cha của một ListView, RecyclerView hoặc GridView. Chú ý là SwipeRefreshLayout chỉ hỗ trợ cho một View đơn, SwipeRefreshLayout không thể chứa vừa RecyclerView vừa GridView được .
main_activity.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <android.support.v4.widget.SwipeRefreshLayout android:id="@+id/srlLayout" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.v7.widget.RecyclerView android:id="@+id/recyclerView" android:layout_width="match_parent" android:layout_height="match_parent"> </android.support.v7.widget.RecyclerView> </android.support.v4.widget.SwipeRefreshLayout> </RelativeLayout>
Tạo thêm custom item cho RecyclerView
cat_name_item.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <TextView android:id="@+id/tvCatName" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:textSize="20sp" /> <View android:layout_width="fill_parent" android:layout_height="1dp" android:background="@android:color/darker_gray" /> </LinearLayout>
Tạo String Array cat names, array này dùng làm dữ liệu để hiển thị lên RecyclerView
<?xml version="1.0" encoding="utf-8"?> <resources> <string-array name="cat_names"> <item>George</item> <item>Zubin</item> <item>Carlos</item> <item>Frank</item> <item>Charles</item> <item>Simon</item> <item>Fezra</item> <item>Henry</item> <item>Schuster</item> <item>Abe</item> <item>Sally</item> <item>Geordie</item> <item>Alex</item> <item>Ebenezer</item> <item>Ulric</item> <item>Donovan</item> </string-array> </resources>
Tạo Custom RecyclerView extends RecyclerView.Adapter
RecyclerViewAdapter.java
package ntc.dev4u.com.swiperefreshlayoutexample; import android.content.Context; import android.support.v7.widget.RecyclerView; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.TextView; import java.util.Arrays; import java.util.Collections; import java.util.List; /** * IDE: Android Studio * Created by Nguyen Trong Cong - NTCDE.COM * Name packge: ntc.dev4u.com.swiperefreshlayoutexample * Name project: SwipeRefreshLayoutExample * Date: 4/13/2017 * Time: 20:43 */ public class RecyclerViewAdapter extends RecyclerView.Adapter<RecyclerViewAdapter.ViewHolder> { List<String> mCatNames; private Context mContext; public RecyclerViewAdapter(Context context) { mContext = context; randomizeCatNames(); } public void randomizeCatNames() { mCatNames = Arrays.asList(getCatNamesResource()); Collections.shuffle(mCatNames); } private String[] getCatNamesResource() { return mContext.getResources().getStringArray(R.array.cat_names); } @Override public ViewHolder onCreateViewHolder(ViewGroup viewGroup, int i) { View inflatedView = LayoutInflater.from(mContext).inflate(R.layout.cat_name_item, viewGroup, false); return new ViewHolder(inflatedView); } @Override public void onBindViewHolder(ViewHolder viewHolder, int i) { String catName = getItem(i); viewHolder.mCatNameTextView.setText(catName); } public String getItem(int position) { return mCatNames.get(position); } @Override public int getItemCount() { return mCatNames.size(); } public class ViewHolder extends RecyclerView.ViewHolder { TextView mCatNameTextView; public ViewHolder(View itemView) { super(itemView); mCatNameTextView = (TextView) itemView.findViewById(R.id.tvCatName); } } }
Cuối cùng là MainActivity
MainActivity.java
package ntc.dev4u.com.swiperefreshlayoutexample; import android.os.Bundle; import android.os.Handler; import android.support.v4.widget.SwipeRefreshLayout; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.LinearLayoutManager; import android.support.v7.widget.RecyclerView; public class MainActivity extends AppCompatActivity implements SwipeRefreshLayout.OnRefreshListener { private RecyclerView mRecyclerView; private SwipeRefreshLayout mSrlLayout; private RecyclerViewAdapter mRecyclerViewAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initView(); } private void initView() { mRecyclerView = (RecyclerView) findViewById(R.id.recyclerView); mSrlLayout = (SwipeRefreshLayout) findViewById(R.id.srlLayout); final LinearLayoutManager layoutManager = new LinearLayoutManager(this); mRecyclerView.setLayoutManager(layoutManager); setupAdapter(); mSrlLayout.setColorSchemeResources(R.color.orange, R.color.green, R.color.blue); mSrlLayout.setOnRefreshListener(this); } @Override public void onRefresh() { new Handler().postDelayed(new Runnable() { @Override public void run() { setupAdapter(); mSrlLayout.setRefreshing(false); } }, 2500); } private void setupAdapter() { mRecyclerViewAdapter = new RecyclerViewAdapter(this); mRecyclerView.setAdapter(mRecyclerViewAdapter); } }
Trong MainActivity bạn thấy method setColorSchemeResources
mSrlLayout.setColorSchemeResources(R.color.orange, R.color.green, R.color.blue);
Phương thức setColorSchemeResources dùng để set màu cho swipe bar, hiển thị khi người dùng vuốt từ trên xuống trong SwipeRefreshLayout.

Sự kiện onRefresh()
@Override public void onRefresh() { new Handler().postDelayed(new Runnable() { @Override public void run() { setupAdapter(); mSrlLayout.setRefreshing(false); } }, 2500); }
Method này sẽ thực hiện hành động khi Refresh Layout, trong hàm trên nó sẽ khởi tạo lại RecyclerViewAdapter ẩn swipe bar sau 2500Millis(2.5s), thường thì phương thức setRefreshing(false) được gọi sau khi đã load xong dữ liệu hoặc load dữ liệu bị lỗi.
Project on Github: https://github.com/trongcong/SwipeRefreshLayoutExample
Cảm ơn bạn ghé thăm blog của mình 😀
Cảm ơn bạn đã chia sẻ